Autoloading Classes
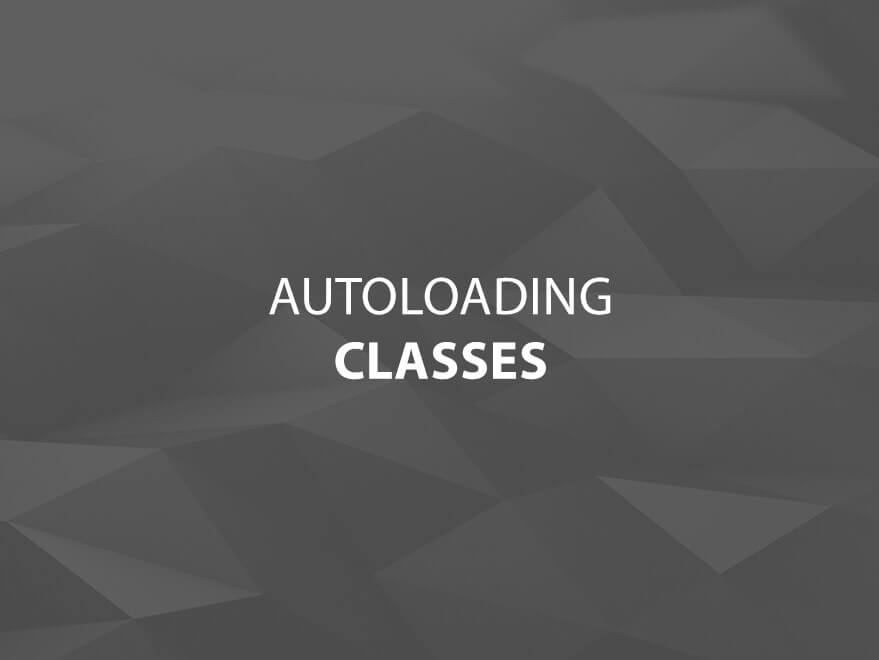
If you’ve done any object oriented programming in PHP, then you are used to creating class files and having to include them in your webpages. There are a couple of ways you can do this. You could include them all in a header file common to all your pages, but if you’ve got a lot of classes, then you may be pulling in a lot of unnecessary code. Alternately, you could just include the class files that you need on each page – but then it becomes a matter of maintenance if you reference a new class on a page, then have to remember to include it. Sure, it’s not a big deal, but there’s another option – class autoloading.
The PHP function spl_autoload_register lets you specify a function to run when you call the classes constructor (i.e. $user = new User();). This function can do anything you want, but most likely it’ll be used to include the file for that class. Below is some example code:
function my_autoloader($class) {
include ‘classes/’ . $class . ‘.class.php’;
}
spl_autoload_register(‘my_autoloader’);
In the example above, spl_autoload_register calls the function my_autoloader. This function has one parameter – the name of the class that is being triggered. It then loads the class file which, in this example, is in the classes folder.
If you are using PHP 5.3 or higher, then you can just define the function as part of the autoload call – for example:
spl_autoload_register(function ($class) {
include ‘classes/’ . $class . ‘.class.php’;
});
All in all, it’s a pretty slick function; it’ll save you some extra lines of code on the top of your pages, and may save you some time in developing.
Comments